Live Field Developer Guide - Javascript
Live Fields are part of a GlobalSearch database’s standard field catalog. To create a Live Field, start as you would when creating any database field. Beginning in GlobalSearch 6.3, an option exists to provision a field as a Live Field.
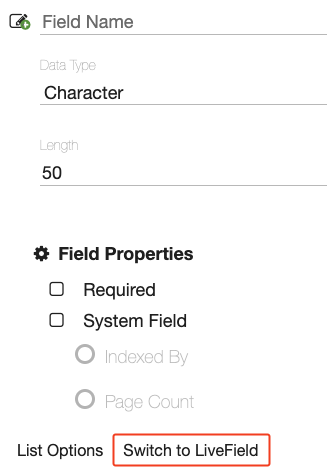
Existing fields can not be converted to Live Fields and vice-versa. This option is only available when creating a new field in the catalog.
Creating a Live Field
The required elements of this field type include a Field Name and some Javascript to execute. Advanced options do exist for making requests to external API sources and databases. For the purposes of this guide, we will focus on Javascript.
Injectable Properties
The Live Fields Javascript interpreter exposes a number of helpers to empower rule building. The elements can be used throughout your Javascript.
$$inject.fields
This property contains a dictionary of getter/setters representing all the fields in the indexer. The values can be accessed by a key of either their field ID or the field's name. i.e. $$inject.fields['Account Name'] = ‘ABC Co.';
will let you set a value to the account name. Setting a value marks the indexer as dirty, so save’s will be allowed.
$$inject.tableFields
This property contains a dictionary of table fields available to the indexer. This is only relevant when the archive has a table field assigned to it.
The table field can be accessed by name as $$inject.tableFields['name of tablefield']
, the table field will have the following properties:
Dictionary of
.columns
, which provides a$$sum
property. i.e.$$inject.tableFields['Line Items'].columns['Amount'].$$sum
.$$rowCount
property to get the number of rows in the table field. Empty rows of data are not included..$$rawData()
function to get a 2D array of the table’s data..$$add(arr)
wherearr
is an array of values to add as a new row, the length ofarr
should match the length of fields/columns in the table. The values will be cast to strings if they are not already..$$clear()
removes all values from the table field.
$$inject.setPendingChanges()
This function will mark the indexer as dirty without requiring a field to be set.
$$inject.save()
This function will invoke a save of the document.
setInterval(function (){ $$inject.save(); }, 60000);
$$inject.properties
A useful set of document and Square9API related properties are exposed for convenience:
.authToken
authentication token..config
exposed viewer application configuration properties object (i.e.; square9ApiUrl, etc.)..document.id
document ID..document.hash
document SecureID search hash..document.databaseId
database ID..document.archiveId
archive ID..document.fileId
viewer cache file ID.
$$inject.notify
A notification service object, provided strings are output to the notification menu (bell icon), containing the following functions
.info()
log an info message..warn()
log an warning message..error()
log an error message..success()
log an success message.
Additionally, a toast message can be shown with .toast()
.
$$inject.notify.toast("This is a viewer toast message.");