Get JSON Node
This node applies to GlobalCapture only.
This node will need to be downloaded from the Square 9 SDN before proceeding.
Node Properties
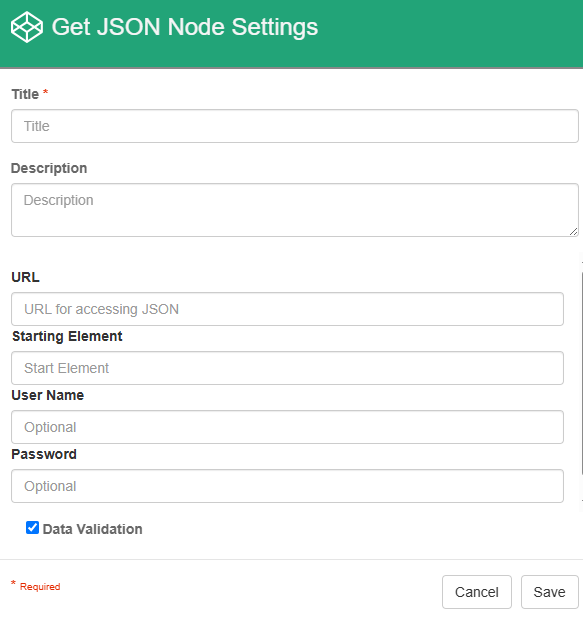
Get JSON Node properties
Title
The Title of your node should be brief but descriptive about what action is being done. Titles are important when revisiting workflows in the future and when migrating workflows. The title of the node will be displayed when resolving conflicts during imports.
Description
The Description of your node should provide notes about this node. This could include information about intended use, details of the connection, etc. Descriptions can be very useful when revisiting workflows in the future.
URL
The URL is the web address to call. S9 notation can also be used to make a dynamic URL.
Example: https://maps.googleapis.com/maps/api/place/findplacefromtext/json?input={p_Web Address}
Starting Element
The Starting Element is the portion of the JSON response that we want to use in our workflow.
Username and Password
The Username and Password are required, as the credentials of the account establishing the connection.
Data Validation
The Data Validation checkbox is enabled by default to enforce Data Types or Length settings for Fields. When enabled, if data does not meet the type or length settings for the field, the process will error on this node.
Action Select
The Get JSON node requires two connections: Success and Failure. If the node successfully connects and returns data, it'll follow the Success path. If the node fails, it'll follow the Failed path.
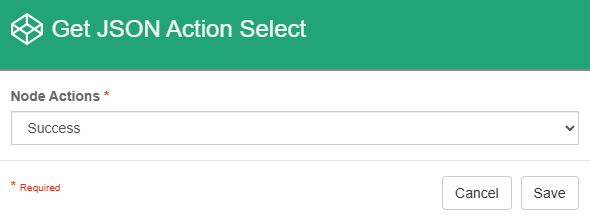
Success Path
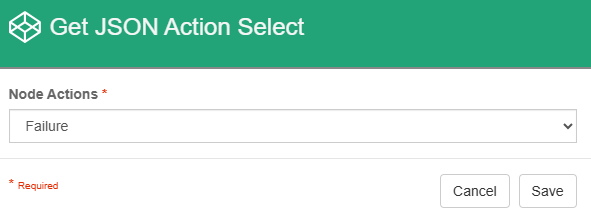
Failure Path
Use Case
In this example, I've configured the GET JSON node to pull back results from Google Places API and output the response as a json format. If a result comes back, it'll proceed down the Success path. If not, it'll proceed down the Failure path, and can be rerouted accordingly.
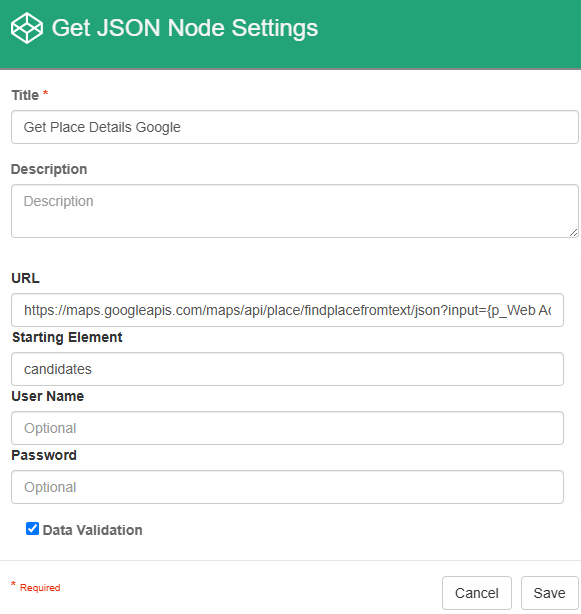
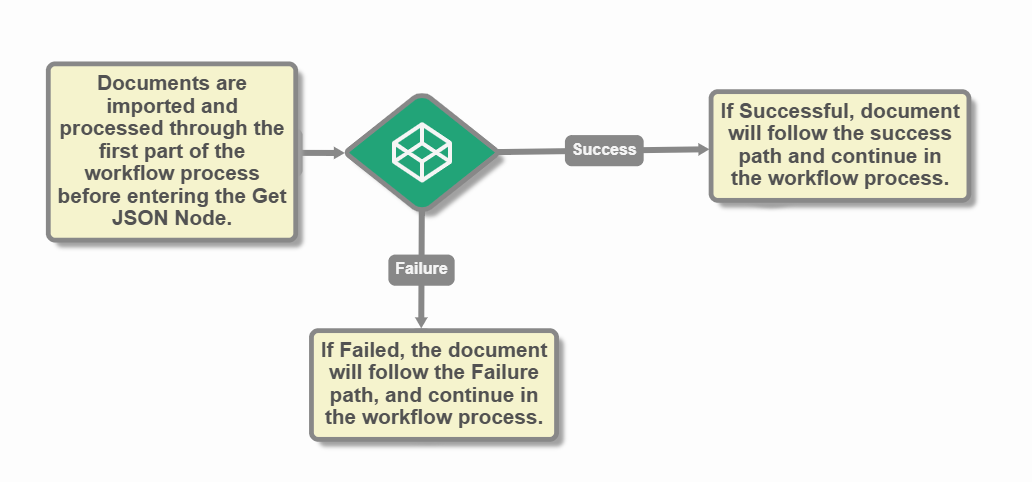
Node Code
public class GetData : CaptureNode //Processing Nodes in GlobalCapture workflows inherit from CaptureNode
{
public override void Run()
{
Uri uri = null;
String root="";
try
{
//Initialize the properties specified at design time. GlobalCapture will already have interpolated any variables.
uri = new Uri(Settings.GetStringSetting("URL"));
root = Settings.GetStringSetting("StartElement");
}
catch
{
//Log any errors to the Process history.
LogHistory("Unable to parse provided URL: " + Settings.GetStringSetting("URL"));
SetNextNodeByLinkName("Failure");
return;
}
IRestResponse response = null;
try
{
//Make the web request.
var client = new RestClient(uri.Scheme + "://" + uri.Authority);
var user = Settings.GetStringSetting("User");
var password = Settings.GetStringSetting("Password");
if (!String.IsNullOrEmpty(user))
{
client.Authenticator = new RestSharp.Authenticators.HttpBasicAuthenticator(user, password);
}
var request = new RestRequest(uri.PathAndQuery, DataFormat.Json);
response = client.Get(request);
if(response.StatusCode != System.Net.HttpStatusCode.OK)
{
throw new Exception("Response received: " + response.StatusCode + ' ' + response.Content);
}
}
catch(Exception ex)
{
LogHistory("Unable to communicate with data source. " + ex.Message);
SetNextNodeByLinkName("Failure");
return;
}
try
{
//Work with the response
dynamic json = JsonConvert.DeserializeObject(response.Content);
var rootObject = json;
if(!string.IsNullOrEmpty(root))
rootObject = json[root];
if (rootObject is JArray)
rootObject = rootObject[0];
if (rootObject == null)
{
LogHistory("No data returned, or no data for key element " + root);
SetNextNodeByLinkName("Failure");
return;
}
//Map elements in the response to matching (by name) Process Fields.
bool propertySet = false;
var propNames = Process.Properties.GetPropertyNames();
foreach (String prop in propNames)
{
String objVal = rootObject[prop];
if (objVal != null)
{
Process.Properties.SetSingleProperty(prop, objVal);
LogHistory(prop + " updating from external data source.");
propertySet = true;
}
}
if(!propertySet)
LogHistory("No data matched cooresponding property.");
SetNextNodeByLinkName("Success");
}
catch(Exception ex)
{
LogHistory("Error parsing response object. " + ex.Message);
SetNextNodeByLinkName("Failure");
return;
}
}
}